Quick Start Guide
1- Setup
Download OPC server and client and set up.
Extract the contents of the zip file. There are two folders for service and client setup. Set up both service and client. You can set up client in computers other than service is set up.
Service is set up as as a Windows service and automatically started. Run client (Softek Data Access Server Manager) is an application.
2- Connect to server
In Data Access Server Manager Press "Connect" button on the toolbar. Enter the server address and press "Connect" button. If your server is located in another computer other than the client is installed then you need to enter IP address of the remote server instead of localhost.
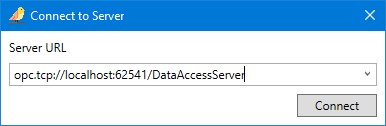
3- Add a controller
Press "New Contoller" button to create a controller in the server address space. Server support Siemens, TwinCat and Omron controllers. Enter a name for the controller. Enter address of the controller reachable from the server. Address is an IP adress for Siemens and Omron. For Twincat it is AMS Net Id (851:5.109.221.24.1.1). For Siemens controllers you need to enter slot number which is normally 2.
When you save the new controller a default block, Block1, is created. Blocks are just collection of tags. You can rename Block1 and create new blocks as required.
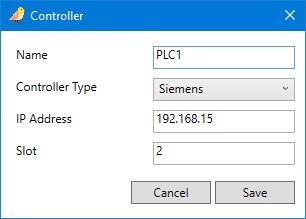
4- Create tags
In the address space tree view click on the block. On the right "Controller tags" pane will be shown. Here, right mouse click and select "New tag". Enter tag information.
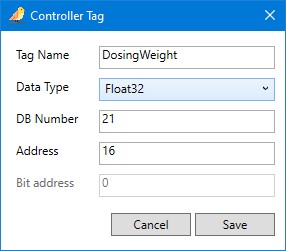
5- Monitor tags
On the created tag right mouse click and select "Monitor tag" to monitor the tag.
The tag will be added to the monitored items pane.
6- Write a program
Here is a program in c# language. Reference SoftekDataAccessClient.exe located in C:\Program Files (x86)\Softek\Softek OPC UA Server Manager directory.
using Softek.Opc.Client;
using System.Windows;
namespace WpfApp1
{
///
/// Interaction logic for MainWindow.xaml
///
public partial class MainWindow : Window
{
OpcUaClient opcClient;
Tag dosingStart;
public MainWindow()
{
InitializeComponent();
opcClient = new OpcUaClient();
opcClient.Connect("opc.tcp://192.168.1.5:62548/Quickstarts/DataAccessServer");
opcClient.CreateTag(this, "PLC1?DosingWeight", DosingWeight_Change);
dosingStart = opcClient.CreateTag(this, "PLC1?DosingStart");
opcClient.ApplyChanges();
}
private void DosingWeight_Change(object tagValue)
{
TextBoxDosingWeight.Text = (string)tagValue;
}
private void Button_Click(object sender, RoutedEventArgs e)
{
dosingStart.Write(true);
}
}
}